Commit ff6a44f
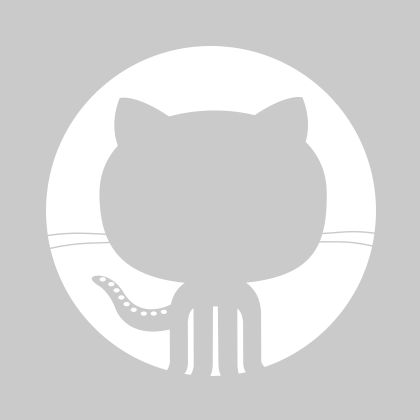
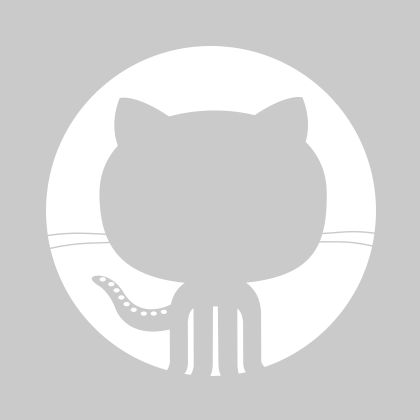
Wu Yu Wei
Stjepan Glavina
1 parent da795de commit ff6a44f
File tree
5 files changed
+60
-66
lines changed- src
- net/driver
- task
5 files changed
+60
-66
lines changed+1-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
33 | 33 |
| |
34 | 34 |
| |
35 | 35 |
| |
36 |
| - | |
37 | 36 |
| |
38 | 37 |
| |
39 | 38 |
| |
40 | 39 |
| |
41 | 40 |
| |
| 41 | + | |
42 | 42 |
| |
43 | 43 |
| |
44 | 44 |
| |
|
+16-18
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 | 3 |
| |
4 |
| - | |
5 | 4 |
| |
| 5 | + | |
6 | 6 |
| |
7 | 7 |
| |
8 | 8 |
| |
| |||
100 | 100 |
| |
101 | 101 |
| |
102 | 102 |
| |
103 |
| - | |
104 |
| - | |
105 |
| - | |
106 |
| - | |
107 |
| - | |
108 |
| - | |
109 |
| - | |
110 |
| - | |
111 |
| - | |
112 |
| - | |
113 |
| - | |
114 |
| - | |
115 |
| - | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
| 109 | + | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
116 | 114 |
| |
117 |
| - | |
| 115 | + | |
| 116 | + | |
118 | 117 |
| |
119 |
| - | |
120 |
| - | |
121 |
| - | |
| 118 | + | |
| 119 | + | |
122 | 120 |
| |
123 | 121 |
| |
124 | 122 |
| |
|
+22-22
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
5 | 5 |
| |
6 | 6 |
| |
7 | 7 |
| |
8 |
| - | |
| 8 | + | |
9 | 9 |
| |
10 | 10 |
| |
11 | 11 |
| |
| |||
19 | 19 |
| |
20 | 20 |
| |
21 | 21 |
| |
22 |
| - | |
23 |
| - | |
24 |
| - | |
25 |
| - | |
26 |
| - | |
27 |
| - | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
28 | 28 |
| |
29 | 29 |
| |
30 | 30 |
| |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
41 |
| - | |
42 |
| - | |
43 |
| - | |
44 |
| - | |
45 |
| - | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
46 | 46 |
| |
47 | 47 |
| |
48 | 48 |
| |
|
+19-21
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
3 | 3 |
| |
4 | 4 |
| |
5 | 5 |
| |
6 |
| - | |
| 6 | + | |
7 | 7 |
| |
8 | 8 |
| |
9 | 9 |
| |
| |||
111 | 111 |
| |
112 | 112 |
| |
113 | 113 |
| |
114 |
| - | |
115 |
| - | |
116 |
| - | |
117 |
| - | |
| 114 | + | |
| 115 | + | |
| 116 | + | |
118 | 117 |
| |
119 |
| - | |
120 |
| - | |
121 |
| - | |
122 |
| - | |
| 118 | + | |
| 119 | + | |
| 120 | + | |
| 121 | + | |
123 | 122 |
| |
124 |
| - | |
125 |
| - | |
126 |
| - | |
127 |
| - | |
128 |
| - | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
| 126 | + | |
| 127 | + | |
129 | 128 |
| |
130 |
| - | |
131 |
| - | |
132 |
| - | |
133 |
| - | |
134 |
| - | |
135 |
| - | |
136 |
| - | |
| 129 | + | |
| 130 | + | |
| 131 | + | |
| 132 | + | |
| 133 | + | |
| 134 | + | |
137 | 135 |
| |
138 | 136 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
5 | 5 |
| |
6 | 6 |
| |
7 | 7 |
| |
8 |
| - | |
| 8 | + | |
9 | 9 |
| |
10 | 10 |
| |
11 | 11 |
| |
| |||
174 | 174 |
| |
175 | 175 |
| |
176 | 176 |
| |
177 |
| - | |
178 |
| - | |
179 |
| - | |
| 177 | + | |
180 | 178 |
| |
181 | 179 |
| |
182 | 180 |
| |
|
0 commit comments